JavaScript language
Parentheses ( )
Parentheses are uses for a few different functions, here are some examples below
Grouping expressions:
Containing expressions:
Containing a list of parameters:
Brackets [ ]
Square brackets provide access to an object’s properties, for example, bracket notion:
Arrays contain a list of data, which is wrapped in square brackets:
Arrays within arrays:
Single quotes '' & Double quotes “”
It important to understand how to use quotes appropriotly in JS. We should use single quotes where you want double quotes to appear inside the string, (e.g. for a html attributes), without having to escape them, or vice versa. Other than that, there is no difference. He is an example.
Either single or double can be used, as long as you are consistant. Below is am example of an object itural, in single quotes:
Either double or single can be used, as long as you are consistant. Example of double quotes:
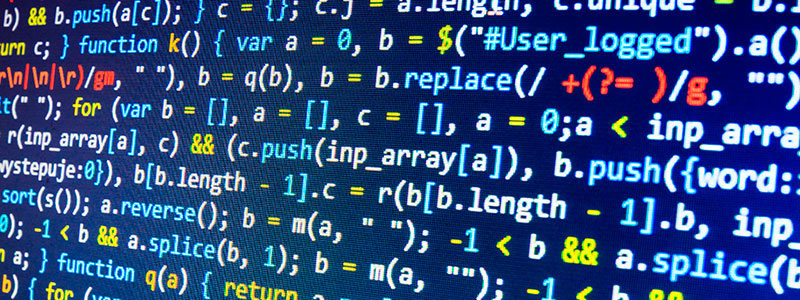