Techniques to get through a technical block
Explain this to a non techie friend....
The question set in this weeks sprint was to explain to a non-tech person how i resolved a problem when i was blocked. There are a bunch of different techniques to use which i have listed below, some more obvious than others, such as googling, researching, calling out to peers and teachers, but this week was the first time i really did some pseudocode.
Pseudocode
Psudocoding is (at a high level) simplifing programming language by turning it into real words that make sense to most people.
This week i was trying to figure out a function example, and i got stuck. I recreated a new function using the same principles, but just in real words or equations that i could understand rather the abbrivations, it worked, then i understood it! By breaking it down into smaller pieces I gained better clarity, and relating real human words to each little bit made it easier to digest.
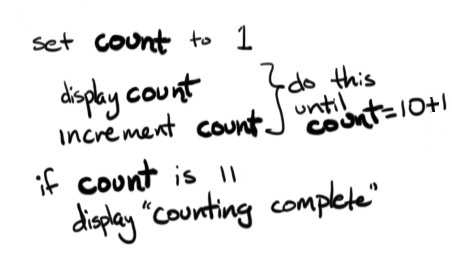
Other problem solving techniques i have tried include;
- Trying something - just having a stab at what you think maybe the answer.
- Reading error messages - editors and dev tools have tons of pointer if you have a good look!
- Console.logging - I haven't done this a huge amount yet but will work on it.
- Googling - Google throws out all kinds of help, how did we manage without it?!
- Asking your peers for help - we probably all can help each other at some point, there is no harm in asking.
- Asking coaches for help - this is after all what our coach are here to do, help and teach.
- Improving your process with reflection - this is definately something to keep working on, to prevent myself making the same mistakes.
What did i learn from this?
I am realising that everything in js code is mostly an abbrieviation of longer code, and what can look quite intimidating when viewed as one big thing is less scary when you break it down into smaller bits. I apply these principles to many things in life, not just code!
.map( )
The official definition of .map is “The map() method creates a new array with the results of calling a provided function on every element in this array.”
In my own understanding this means i can run some function against an array and map() will return the results in a new array. In real life perhaps this method is useful for applying a discount to a customers normal bill…
.filter( )
The official definition of .filter is “The filter() method creates a new array with all elements that pass the test implemented by the provided function.”
In my own words filter does what it says on the tin, it will run a function against an array and create a new array that passes the conditions in that function. In real life perhaps we want a list of students of exam results over a certain value….
.reduce( )
The official definition of .reduce() is "The reduce() method applies a function against an accumulator and each value of the array (from left-to-right) to reduce it to a single value."
You can use this function to flatten an array of arrays, for example, reduce() could convert myArr = [[0, 1], [2, 3], [4, 5]] into [0, 1, 2, 3, 4, 5]. I’m still trying to understand what this methods is used for in real life….