How does JavaScript compare to HTML and CSS?
JS is one in a trio of coding languages that can join together to form one webpage. HTML is our basic structure of the site, creating paragraphs, blocks and tables etc, and CSS styles the site. The main function of JS is to tell the browser how to response to interactions from the user, mostly from inputing data. A function in JS takes in inputs, does something with them, and produces an output.
Control flow and loops
JS supports flow statements like if, for, and while. Here are a few analogies to help explain in everyday examples:
IF I have coffee THEN have 2 sugars, otherwise just have tea.
WHILE i am running, i want endemondo to time me and track my pace, but as soon as i stop running i want it to stop tracking my time and pace.
A For loop already know when to stop at some point in time.
For example, when running, i want endemondo to log the timer, every 1km so i know my time - but i want it to stop timing when i stop running.
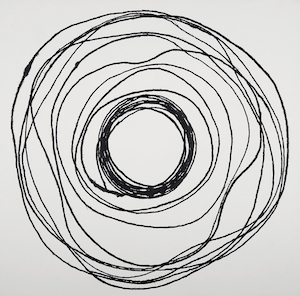
Explain the difference between accessing data from arrays and object literals.
An array is a special variable, which can hold more than one value at a time.You refer to an array element by referring to the index number.
Accessing data from arrays:
Array:
To access data we use bracket [ ] notation and refer to index:
Accessing data from literals:
An object literal is a comma-separated list of name-value pairs wrapped in curly braces. Object literals encapsulate data, enclosing it in a tidy package. The property values can be of any data type, including array literals, functions, and nested object literals.
Object:
To access data we can use literal notation: